AndroidはPreferenceActivityで簡単に設定画面を作ることができる。
数種類の設定項目があるけど色を設定する項目はないのでシンプルなものを作ってみた。
src/jp/playground/PrefActivity.java 設定画面本体
package jp.playground;
import android.content.Context;
import android.graphics.Color;
import android.os.Bundle;
import android.preference.PreferenceActivity;
import android.preference.PreferenceManager;
public class PrefActivity extends PreferenceActivity {
private static final String KEY_COL1 = "col1";
private static final int DEF_COL1 = Color.rgb(0, 0, 0);
RgbDialogPreference col1;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
addPreferencesFromResource(R.xml.pref);
//色設定項目を探してデフォルト値設定
col1 = (RgbDialogPreference)findPreference(KEY_COL1);
col1.setDefault(DEF_COL1);
}
//色設定値を取得
public static int getCol1(Context con) {
return PreferenceManager.getDefaultSharedPreferences(con).getInt(
KEY_COL1, DEF_COL1);
}
}
res/xml/pref.xml 設定のリソース
<?xml version="1.0" encoding="utf-8"?>
<PreferenceScreen
xmlns:android="http://schemas.android.com/apk/res/android">
<PreferenceCategory
android:selectable="true"
android:enabled="true"
android:title="@string/PrefCategoryColor">
<jp.playground.RgbDialogPreference
android:selectable="true"
android:enabled="true"
android:title="@string/PrefColor1"
android:key="col1">
</jp.playground.RgbDialogPreference>
</PreferenceCategory>
</PreferenceScreen>
src/jp/playground/RgbDialogPreference.java RGB設定ダイアログ
DialogPreferenceから派生している
ダイアログ内の各要素はonBindDialogViewで渡ってくるviewから取得している
package jp.playground;
import android.content.Context;
import android.content.SharedPreferences;
import android.content.SharedPreferences.Editor;
import android.graphics.Color;
import android.preference.DialogPreference;
import android.preference.PreferenceManager;
import android.util.AttributeSet;
import android.view.View;
import android.widget.SeekBar;
public class RgbDialogPreference extends DialogPreference implements SeekBar.OnSeekBarChangeListener {
private static SharedPreferences sp;
private SeekBar barR;
private SeekBar barG;
private SeekBar barB;
private ViewColor cView;
private int defaultColor = 0;
public RgbDialogPreference(Context context, AttributeSet attrs) {
super(context, attrs);
sp = PreferenceManager.getDefaultSharedPreferences(context);
// ダイアログのレイアウトリソース指定
setDialogLayoutResource(R.layout.rgbdlg);
}
@Override
protected void onBindDialogView(View v) {
super.onBindDialogView(v);
barR = (SeekBar)v.findViewById(R.id.SeekBarRed);
barR.setOnSeekBarChangeListener(this);
barG = (SeekBar)v.findViewById(R.id.SeekBarGreen);
barG.setOnSeekBarChangeListener(this);
barB = (SeekBar)v.findViewById(R.id.SeekBarBlue);
barB.setOnSeekBarChangeListener(this);
//色表示用View
cView = (ViewColor)v.findViewById(R.id.ColorView);
barR.setProgress(Color.red(getVal()));
barG.setProgress(Color.green(getVal()));
barB.setProgress(Color.blue(getVal()));
update();
}
@Override
protected void onDialogClosed(boolean positiveResult) {
if (positiveResult) {
setVal(Color.rgb(barR.getProgress(), barG.getProgress(), barB.getProgress()));
}
}
@Override
public void onProgressChanged(SeekBar seekBar, int progress,
boolean fromUser) {
// TODO Auto-generated method stub
update();
}
@Override
public void onStartTrackingTouch(SeekBar seekBar) {
// TODO Auto-generated method stub
}
@Override
public void onStopTrackingTouch(SeekBar seekBar) {
// TODO Auto-generated method stub
}
private void setVal(int value) {
Editor e = sp.edit();
e.putInt(getKey(), value);
e.commit();
}
private int getVal() {
return sp.getInt(getKey(), defaultColor);
}
/* 表示更新 */
private void update() {
cView.setColor(Color.rgb(barR.getProgress(), barG.getProgress(), barB.getProgress()));
cView.invalidate();
}
public void setDefault(int col) {
defaultColor = col;
}
}
res/layout/rgbdlg.xml RGB設定ダイアログのリソース
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="horizontal"
android:layout_height="wrap_content"
android:layout_width="wrap_content"
android:gravity="center"
android:paddingBottom="5dp">
<LinearLayout
android:orientation="vertical"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_vertical|center_horizontal"
android:layout_marginLeft="5dp"
android:layout_marginRight="10dp">
<jp.playground.ViewColor
android:id="@+id/ColorView"
android:layout_width="70dp"
android:layout_height="70dp"
android:paddingLeft="30dp"
android:paddingRight="30dp">
</jp.playground.ViewColor>
</LinearLayout>
<LinearLayout
android:orientation="vertical"
android:layout_width="wrap_content"
android:layout_height="wrap_content">
<TextView android:id="@+id/TextView01"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="@string/DlgRgbRed"
android:layout_gravity="center_horizontal">
</TextView>
<SeekBar android:id="@+id/SeekBarRed"
android:layout_height="wrap_content"
android:max="255"
android:layout_width="150dp"
android:paddingLeft="10px"
android:paddingRight="10px">
</SeekBar>
<TextView android:id="@+id/TextView02"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="@string/DlgRgbGreen"
android:layout_gravity="center_horizontal">
</TextView>
<SeekBar android:id="@+id/SeekBarGreen"
android:layout_height="wrap_content"
android:max="255"
android:layout_width="150dp"
android:paddingLeft="10px"
android:paddingRight="10px">
</SeekBar>
<TextView android:id="@+id/TextView03"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="@string/DlgRgbBlue"
android:layout_gravity="center_horizontal">
</TextView>
<SeekBar android:id="@+id/SeekBarBlue"
android:layout_height="wrap_content"
android:max="255"
android:layout_width="150dp"
android:paddingLeft="10px"
android:paddingRight="10px">
</SeekBar>
</LinearLayout>
</LinearLayout>
src/jp/playground/ViewColor.java 色を表示するだけのView
package jp.playground;
import android.content.Context;
import android.graphics.Canvas;
import android.graphics.Paint;
import android.graphics.RectF;
import android.util.AttributeSet;
import android.view.View;
public class ViewColor extends View {
public ViewColor(Context context, AttributeSet attrs) {
super(context, attrs);
// TODO Auto-generated constructor stub
}
int colBG = 0;
Paint paint = new Paint();
RectF rbase = new RectF();
@Override
protected void onDraw (Canvas canvas)
{
rbase.set(0, 0, getWidth(), getHeight());
paint.setColor(colBG);
canvas.drawRect(rbase, paint);
}
public void setColor(int col)
{
colBG = col;
}
}
src/jp/playground/playground.java 呼び出し元のActivity
package jp.playground;
import android.app.Activity;
import android.content.Intent;
import android.os.Bundle;
import android.view.Menu;
import android.view.MenuInflater;
import android.view.MenuItem;
public class playground extends Activity {
private static final int SHOW_PREFERENCE = 1;
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
}
/* メニュー内容を生成 */
@Override
public boolean onCreateOptionsMenu(Menu menu) {
MenuInflater inflater = getMenuInflater();
inflater.inflate(R.menu.menu, menu);
return true;
}
/* ボタンを押した時の動作 */
@Override
public boolean onMenuItemSelected(int featureId, MenuItem item) {
int id = item.getItemId();
// XML中のメニューボタンにアクセスするにはR.id以下を利用する
if (id == R.id.setting) {
Intent i = new Intent(getApplicationContext(), PrefActivity.class);
startActivityForResult(i,SHOW_PREFERENCE);
}
return true;
}
/* PrefActivityから戻った */
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
if (requestCode == SHOW_PREFERENCE) {
//設定値取得
int col1 = PrefActivity.getCol1(getApplicationContext());
}
}
}
こんな画面
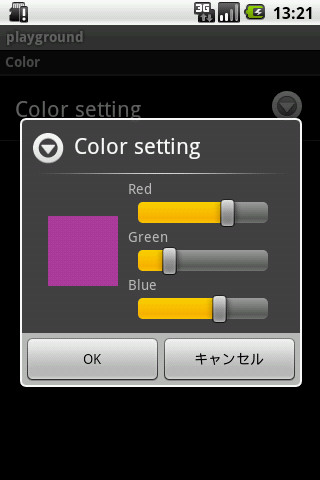
コメントを残す